Automation inputs
This page will present examples and details about each type of automation inputs. For most input types, there are examples of how to define inputs that take single or multiple values. Furthermore, the examples show how to define inputs that are required, but you can also learn how to make any input optional.
Prerequisite
At the beginning of your automation code, you will need to import the available (and needed) input and output types. Primitive types such as strings and integers are supported natively in Python, while the ones that are related to the Seven Bridges environment need to be imported from hephaestus
as follows:
from hephaestus.types import File, Folder, VolumeFile, VolumeFolder, Task, Project
String
This input object represents a string value and its type is str
. When rendered in the visual interface, strings are represented as single-line text inputs that take values of up to 2000 characters.
Example:
string_input = Input(
str,
name='String input',
description='A string input.',
default='Default string'
)
Attributes:
name
- String. Name of the string input that will be shown in the visual interface.description
- String. Text description that provides more details about the string input. Displayed as a hint next to the input.default
- String. Default value that will be used if no value is provided on the input.
Visual interface:
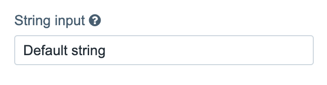
Multi-string inputs
To be able to pass multiple string values on the same input, the type of input in the example above needs to be changed to List[str]
. As this input type does not have a dedicated component in the visual interface yet, see how such input types are handled.
Boolean
Boolean input whose type is defined as bool
. Possible values can be Null
, True
or False
.
Example:
boolean_input = Input(
bool,
name='Boolean input',
description='This is a boolean input.',
default=False
)
Attributes:
name
- String. Name of the boolean input that will be shown in the visual interface.description
- String. Text description that provides more details about the boolean input. Displayed as a hint next to the input.default
- Boolean. Default value that will be used if no value is provided on the input.
Visual interface:
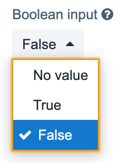
Multiple boolean values on a single input
To be able to pass multiple boolean values on the same input, the type of input in the example above needs to be changed to List[bool]
. As this input type does not have a dedicated component in the visual interface yet, see how such input types are handled.
Integer
Integer input whose type is defined as int
. Allows negative values and does not allow values like 0.3 as they are considered as a float. The range of integer values is limited only by available memory.
Example:
integer_input = Input(
int,
name='Integer input',
description='This is an integer input.',
default=1
)
Attributes:
name
- String. Name of the integer input that will be shown in the visual interface.description
- String. Text description that provides more details about the integer input. Displayed as a hint next to the input.default
- Integer. Default value that will be used if no value is provided on the input.
Visual interface:
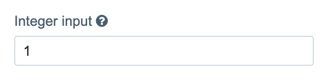
Multiple integer values on a single input
To be able to pass multiple integer values on the same input, the type of input in the example above needs to be changed to List[int]
. As this input type does not have a dedicated component in the visual interface yet, see how such input types are handled.
Float
Float input whose type is defined as float
.
Example:
float_input = Input(
float,
name='Float input',
description='This is a float input.',
default=0.3
)
Attributes:
name
- String. Name of the float input that will be shown in the visual interface.description
- String. Text description that provides more details about the float input. Displayed as a hint next to the input.default
- Float. Default value that will be used if no value is provided on the input.
Visual interface:
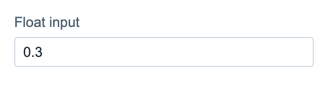
When providing a value for a float input through the visual interface, you can use either decimal or scientific notation.
Multiple float values on a single input
To be able to pass multiple boolean values on the same input, the type of input in the example above needs to be changed to List[float]
. As this input type does not have a dedicated component in the visual interface yet, see how such input types are handled.
Enum
Enums are a set of symbolic names (members) bound to unique, constant values. In the context of automation inputs in the visual interface, they are displayed as drop-down lists.
When developing automations, definition of enums as inputs involves creation of an enumeration using the class
syntax and, subsequently, definition of an Enum input.
Prerequisite - import the Enum
and NamedMember
classes from freyja
:
from freyja import Enum
from freyja.types import NamedMember
Create an enumeration following the syntax in the example:
class Enumeration(Enum):
EN1 = NamedMember(value=1, name='Value 1')
EN2 = NamedMember(value=my_object, name='Value 2')
EN3 = 5
EN4 = my_object_2
Enumeration members can be defined in the following ways:
- By assigning primitive values, such as strings or integers, directly to enumeration members, for example
EN3 = 5
. The visual interface will display the value, which is5
in this case, in the Enum input dropdown in the visual interface. - Using
NamedMember
, following theMEMBER = NamedMember(value=<member_value>, name='Member name')
syntax. This approach allows you to assign either primitive or complex types (such as objects) as member values, but also to use the name property to define custom descriptive member names that will appear in the dropdown in the visual interface. - By assigning complex values directly to enumeration members, for example
EN4 = my_object_2
. This assigns the object namedmy_object_2
to theEN4
member and results in displayingEN4
in the Enum input dropdown in the visual interface.
Finally, define an enum input by using the class defined above as the input type:
enum_input = Input(
Enumeration,
name='Enum type input',
description='One enum input with default value' ,
default = Enumeration.EN1
)
Attributes:
name
- String. Name of the enum input that will be shown in the visual interface.description
- String. Text description that provides more details about the enum input. Displayed as a hint next to the input.default
- Enumeration member. Default value that will be used if no value is provided for the enum input. Defined as<EnumerationClass>.<enumeration_member>
.
Visual interface:
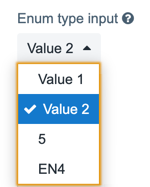
When writing automation code, value of an Enum input can be retrieved using the self.enum_input.value
syntax.
File
This input object represents a Platform file and is rendered as a file picker in the visual interface.
Example:
file_input = Input(
File,
name='Single file',
description='Should open file-picker in projects tab at '
'rfranklin/release-project:inner_folder, does '
'not allow to select folders and allows only single selection',
default='567890abc9b0307bc0414164',
meta={'location': 'rfranklin/release-project:inner_folder',
'types': ['.bam', '.zip']}
)
Attributes:
name
- String. Name of the File input that will be shown in the visual interface.description
- String. Text description that provides more details about the File input. Displayed as a hint next to the input.default
- String. ID of the file that will be used by default if no value is provided on the input. File ID is available in the URL when viewing file details through the Platform's visual interface, or when listing files in a project through the API.meta
- Dictionary. Additional information related to the File input:location
- String. The default location where the file picker opens, defined in the following format:- For the Public Files gallery:
'location': 'public'
- For a specific project on the Platform:
'location': 'username/project-name'
- For a subdirectory within a project:
'location': 'username/project-name:subdirectory'
- For the Public Files gallery:
types
- List of strings. One or more extensions that are be applied as a filter in the file picker, entered as strings, including the dot:'.bam'
Providing files input values through the CLI
When running an automation through the command-line interface, use the following syntax to specify a file as the input:
sb automations start --package-id <id> --inputs '{"file_input": <file-id>}'
Make sure to replace <file-id>
with the ID of the folder you want to use as the input.
Selecting multiple files
To allow selection of multiple files in the file picker, the type of input in the example above needs to be changed to List[File]
as shown below:
multiple_files_input = Input(
List[File],
description='This allows you to select multiple files',
default=['567890abc9b0307bc0414164','567890abc1e5339df0414123'],
name='Multiple files',
meta={'location': 'rfranklin/release-project:inner_folder',
'types': ['.bam', '.zip']}
)
Multiple file inputs have the same attributes as single file inputs. Please note this will allow you to select Platform files only. To be able to select files from an attached volume, please see the VolumeFile input type. Platform and Volume files can never be combined on the same input.
Folder
This input type represents a Platform folder and is rendered as a file picker in the visual interface. The picker will allow you to select folder(s) only.
Example:
folder_input = Input(
Folder,
name='Single folder',
description='Opens the file picker at the location defined below',
default='567890abc9b0307bc0414164',
meta={'location': 'rfranklin/release-project'}
)
Attributes:
name
- String. Name of the Folder input that will be shown in the visual interface.description
- String. Text description that provides more details about the Folder input. Displayed as a hint next to the input.default
- String. ID of the folder that will be used by default if no value is provided on the input. Folder ID can be obtained via the API when listing files and folders within a project:
curl -s -H "X-SBG-Auth-Token: <your-api-token-here>" -H "content-type: application/json" -X GET "https://api.sbgenomics.com/v2/files?project=<username/slug>"
Or you can get the ID of a folder directly by using the same call with the addition of the name
parameter that specifies the name of the folder:
curl -s -H "X-SBG-Auth-Token: <your-api-token-here>" -H "content-type: application/json" -X GET "https://api.sbgenomics.com/v2/files?project=<username/slug>&name=<folder-name>"
Learn more about listing files in a project via the API.
meta
- Dictionary. Additional information related to the Folder input:location
- String. The default location where the folder picker opens, defined in the following format:- For a specific project on the Platform:
'location': 'username/project-name'
- For a subdirectory within a project:
'location': 'username/project-name:subdirectory'
- For a specific project on the Platform:
Providing folder input values through the CLI
When running an automation through the command-line interface, use the following syntax to specify a folder as the input:
sb automations start --package-id <id> --inputs '{"folder_input": <folder-id>}'
Make sure to replace <folder-id>
with the ID of the folder you want to use as the input.
Selecting multiple folders
To allow selection of multiple folders in the file picker, the type of input in the example above needs to be changed to List[Folder]
as shown below:
folder_input = Input(
List[Folder],
name='Multiple folders',
description='Opens the file picker at the location defined below',
default=['567890abc9b0307bc0414164','567890abc1e5339df0414123'],
meta={'location': 'rfranklin/release-project'}
)
Multiple folder inputs have the same attributes as single folder inputs. Please note this will allow you to select Platform folders only. To be able to select folders from an attached volume, see the VolumeFolder input type. Platform and Volume folders can never be combined on the same input.
VolumeFile
This input object represents a file from an attached volume and is rendered as a file picker in the visual interface. As with Platform files, you can define a single or multiple file inputs, as shown in the paragraphs below.
Example:
volume_file_input = Input(
VolumeFile,
name='Volume file',
description='Should open file-picker at '
'rfranklin/attached_volume:subdirectory, does '
'not allow to select folders and allows only single selection',
default='rfranklin/attached_volume:subfolder/volume_file.ext',
meta={'location': 'rfranklin/attached_volume:subfolder'}
)
Attributes:
name
- String. Name of the VolumeFile input that will be shown in the visual interface.description
- String. Text description that provides more details about the VolumeFile input. Displayed as a hint next to the input.default
- String. Path to the file that will be used by default if no value is provided on the input. The path has the following format:<username>/<volume_name>:<volume_folder>/<volume_file>
.meta
- Dictionary. Additional information related to the VolumeFile input:location
- String. The default location where the file picker opens, defined in the following format:- For the root folder of an attached volume:
'location': 'username/volume-name'
- For a subfolder within a volume:
'location': 'username/volume-name:subfolder'
- For the root folder of an attached volume:
Providing volume file input values through the CLI
When running an automation through the command-line interface, use the following syntax to specify a volume file as the input:
sb automations start --package-id <id> --inputs '{"volume_file": "<volume_owner>/<volume_name>:<subfolder>/<file.ext>"}'
Make sure to replace all placeholders in the volume file path above to match the volume and file location in your specific use case.
Selecting multiple volume files
To allow selection of multiple volume files in the file picker, the type of input in the example above needs to be changed to List[VolumeFile]
as shown below:
volume_file_input = Input(
List[VolumeFile],
name='Volume files',
description='Should open file-picker at '
'rfranklin/attached_volume:subdirectory, does not '
'allow selection of folders and allows multiple selection',
default=['567890abc9b0307bc0414164','567890abc1e5339df0414123'],
meta={'location': 'rfranklin/attached_volume:subdirectory'}
)
Please note this will allow you to select Volume files only. To be able to select files from the Platform, see the File input type. Platform and Volume files can never be combined on the same input.
VolumeFolder
This input object represents a folder from an attached volume and is rendered as a file picker in the visual interface. As with Platform folders, you can define a single or multiple folder inputs, as shown in the paragraphs below.
Example:
volume_folder_input = Input(
VolumeFolder,
name='Input for a single volume folder',
description='Allows selection of a single folder only',
default='rfranklin/attached-volume:folder',
meta={'location': 'rfranklin/attached_volume:subfolder'}
)
Attributes:
name
- String. Name of the VolumeFile input that will be shown in the visual interface.description
- String. Text description that provides more details about the VolumeFile input. Displayed as a hint next to the input.default
- String. Path to the volume folder that will be used by default if no value is provided. The path has the following format:'username/volume-name:folder-name'
meta
- Dictionary. Additional information related to the VolumeFolder input:location
- String. The default location where the file picker opens, defined in the following format:- For the root folder of an attached volume:
'location': 'username/volume-name'
- For a subfolder within a volume:
'location': 'username/volume-name:subfolder'
- For the root folder of an attached volume:
Providing volume folder input values through the CLI
When running an automation through the command-line interface, use the following syntax to specify a volume folder as the input:
sb automations start --package-id <id> --inputs '{"volume_folder": "<volume_owner>/<volume_name>:<subfolder>"}'
Make sure to replace all placeholders in the volume folder path above to match the volume and folder location in your specific use case.
Selecting multiple volume folders
To allow selection of multiple volume folders in the file picker, the type of input in the example above needs to be changed to List[VolumeFolder]
as shown below:
volume_folders_input = Input(
List[VolumeFolder],
description='Allows selection of multiple volume folders',
name='Input for volume folders',
default=['rfranklin/attached-volume:folder'],
meta={'location': 'rfranklin/attached-volume'}
)
Multiple VolumeFolder
inputs have almost identical attributes as single folder inputs. The only difference is in the default
attribute that takes a list of strings (volume folder paths) instead of a single string (path).
Please note this will allow you to select Volume folders only. To be able to select folders from the Platform, see the Folder input type. Platform and Volume folders can never be combined on the same input.
Project
This input type represents a project on the Platform. The visual interface renders it as a project picker and the value returned is the slug of the selected project (user-name/project-name
).
Example:
project_input = Input(
Project,
name='Project input',
description='Allows selection of a Platform project.',
default='rfranklin/my-project'
)
Attributes:
name
- String. Name of the Project input that will be shown in the visual interface.description
- String. Text description that provides more details about the Project input. Displayed as a hint next to the input.default
- String. Project slug that will be used by default if no value is provided. The slug has the following format:'username/project-name'
Visual interface:
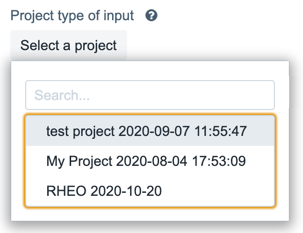
Selection of multiple projects is currently not supported within standard automation inputs.
Defining the Project input for project-based automations
When developing project-based automations, your code packages must contain a specific Project input named sb_project_id
in order for the functionality of binding automations to a single project to work. Here's an example of a properly defined sb_project_id
input:
sb_project_id = Input(Project)
Along with defining the automation as project-based when it is created, this will allow the visual interface will remember your current project and constrain the scope of the automation to that project.
Making inputs optional
Any input can be defined as optional, meaning that it will be possible to run the automation without providing a value for the specific input. If needed, you can also provide a default value for an optional input.
To make an input optional, first make sure that the Optional
class is imported from Freyja at the beginning of your automation code:
from freyja import Optional
To make an input optional, use Optional[<input-type>]
as shown below:
file_input = Input(
Optional[File],
name='Optional file input',
description='This is an optional file input',
default='567890abc1e5339df0414123'
)
Optional
can also be used with list-type inputs that take multiple values, using nesting that follows the Optional[List[<input-type>]]
pattern, as shown below:
file_input = Input(
Optional[List[File]],
name='Optional file input',
description='This is an optional file input.'
)
Optional inputs will be rendered in the Optional inputs and settings dropdown section in the visual interface.
Checking if an optional input has a value
An important consideration when working with optional inputs is how to check whether there is a value provided on the input. The simple Pythonic check using the is
operator and checking whether the value is None
(e.g. optional_string_input is None
) will not provide the desired result as it checks whether the promise object itself is None
, which is never the case. Instead, try using the ==
and !=
operators which will actually test whether a value is available or not.
Handling input types that are not supported by the visual interface
Rendering of automation inputs on the visual interface happens by displaying adequate UI controls for the defined input types. However, if the automation code contains an input type that is valid but does not have an adequate UI component, it will be rendered as a text box and you can provide a JSON-formatted value to it.
The following input types don't have dedicated in the visual interface:
- List of strings (
List[str]
) - List of integers (
List[int]
) - List of floats (
List[float]
) - List of boolean values (
List[bool]
)
For example, this is the code of an input that does not have a dedicated component in the visual interface:
unsupported = Input(
List[str],
name='List of strings input',
description='This is not supported by the GUI.',
default=["a", "b"]
)
When rendered in the visual interface, input types that do not have a dedicated component are displayed as a text field by default:
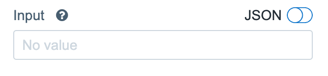
If the automation code expects a primitive value type on the input, such as a string, it is enough to enter the value in the displayed text field. When using this option, the entered value is passed verbatim as primitive str
type to the underlying automation input. However, if a more complex value needs to be provided, use the JSON toggle in the top-right corner to switch to the multi-line input box and enter your JSON-formatted value:

Valid formatting of a JSON input in case of input types that are not supported by the visual interface is a brackets-enclosed object-like value or list of values, with the usage of double quotes only. The keys of the object-like value are always primitives (e.g. a string or number) enclosed in quotes, whereas the value can be a dictionary, list, or a primitive.
Examples:
["one", 2, "three"]
{
"one": "string",
"2": ["2", "four"],
"three": {
...
}
}
Updated almost 3 years ago